Installation
Open Terminal and install it by homebrew.$
ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)" < /dev/null 2> /dev/null
$ brew install ranger
$ ranger --copy-config=all
After installing, type $ ranger to play around.
Navigation
Ranger uses many of the same keybindings as "vim". The way of navigation is similar to VIM. Below are some basic hotkeys for your reference.
Screenshot
Then, paste the below snippet to map the below commands to hotkeys.
$ vim ~/.config/ranger/rc.conf
Second, create a plugin for that. After finishing this step, you can open ranger again, then type 'cj' to execute the autojump.
j = Move down
k = Move up
h = Move to parent directory
l = Go to the next level
gg = Go to the top of the list
G = Go to the bottom of the list
tab + n = Create a new tab
tab = Switch between differernt tabs
For more hotkey information, you can reference here
Basic file operation
D = delete files
i = Display file (useful if you'd like to view a text file in a pager instead of editing it)
l or E = Open file (opens file in default file-handler)
r = Open file with… (allows you to choose program to use)
o = Change sort order (follow by character in menu selection)
z = Change settings (commonly used toggle settings)
zh = View hidden files
<space> = Select current file
t = Tag file (you can perform actions on tagged files)
cw = Rename current file
/ = Search for files
n = Jump to next match
N = Jump to previous match
yy = Yank (copy) file
dd = Mark file for cut operation
<delete> = Delete selected file
As a side note, if you would like to avoid deleting files or folder permanently by mistake, you can consider having trash-cli.
$ npm install --global trash-cli # install trash-cli
Hook up and override delete functionality in Ranger. Map 'DD' key to delete to Trash by adding this to your ~/.config/ranger/rc.conf
$ vim ~/.config/ranger/rc.conf
Add the below line to rc.conf
map DD shell trash %s
Preview images in Ranger
Add the below two lines to rc.conf
set preview_images true set preview_images_method iterm2
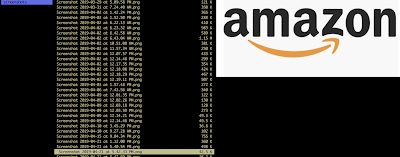
Integration with fzf
$ vim ~/.config/ranger/commands.py
from ranger.api.commands import Command
# https://github.com/ranger/ranger/wiki/Integrating-File-Search-with-fzf
# Now, simply bind this function to a key, by adding this to your ~/.config/ranger/rc.conf: map <C-f> fzf_select
class fzf_select(Command):
"""
:fzf_select
Find a file using fzf.
With a prefix argument select only directories.
See: https://github.com/junegunn/fzf
"""
def execute(self):
import subprocess
if self.quantifier:
# match only directories
command="find -L . \( -path '*/\.*' -o -fstype 'dev' -o -fstype 'proc' \) -prune \
-o -type d -print 2> /dev/null | sed 1d | cut -b3- | fzf +m"
else:
# match files and directories
command="find -L . \( -path '*/\.*' -o -fstype 'dev' -o -fstype 'proc' \) -prune \
-o -print 2> /dev/null | sed 1d | cut -b3- | fzf +m"
fzf = self.fm.execute_command(command, stdout=subprocess.PIPE)
stdout, stderr = fzf.communicate()
if fzf.returncode == 0:
fzf_file = os.path.abspath(stdout.decode('utf-8').rstrip('\n'))
if os.path.isdir(fzf_file):
self.fm.cd(fzf_file)
else:
self.fm.select_file(fzf_file)
Then, paste the below snippet to map the below commands to hotkeys. from ranger.api.commands import Command # https://github.com/ranger/ranger/wiki/Integrating-File-Search-with-fzf # Now, simply bind this function to a key, by adding this to your ~/.config/ranger/rc.conf: map <C-f> fzf_select class fzf_select(Command): """ :fzf_select Find a file using fzf. With a prefix argument select only directories. See: https://github.com/junegunn/fzf """ def execute(self): import subprocess if self.quantifier: # match only directories command="find -L . \( -path '*/\.*' -o -fstype 'dev' -o -fstype 'proc' \) -prune \ -o -type d -print 2> /dev/null | sed 1d | cut -b3- | fzf +m" else: # match files and directories command="find -L . \( -path '*/\.*' -o -fstype 'dev' -o -fstype 'proc' \) -prune \ -o -print 2> /dev/null | sed 1d | cut -b3- | fzf +m" fzf = self.fm.execute_command(command, stdout=subprocess.PIPE) stdout, stderr = fzf.communicate() if fzf.returncode == 0: fzf_file = os.path.abspath(stdout.decode('utf-8').rstrip('\n')) if os.path.isdir(fzf_file): self.fm.cd(fzf_file) else: self.fm.select_file(fzf_file)
$ vim ~/.config/ranger/rc.conf
map cf fzf_select map cl fzf_locateNow, try to open Ranger, and type `cf`. It will show fzf search console
Integration with autojump
With integration with autojump, it makes you faster to navigate your filesystem.
First, create rc.conf in ~/.config/ranger
$ vim ~/.config/range/rc.conf
Paste the key mapping between the below plugin and hotkey.
map cj console j%space
$ mkdir -p ~/.config/ranger/plugins $ vim ~/.config/ranger/plugins/autojump.pyPaste the below code to autojump.py. You can reference my settings and code here.
import ranger.api import subprocess from ranger.api.commands import * HOOK_INIT_OLD = ranger.api.hook_init def hook_init(fm): def update_autojump(signal): subprocess.Popen(["autojump", "--add", signal.new.path]) fm.signal_bind('cd', update_autojump) HOOK_INIT_OLD(fm) ranger.api.hook_init = hook_init class j(Command): """:j Uses autojump to set the current directory. """ def execute(self): directory = subprocess.check_output(["autojump", self.arg(1)]) directory = directory.decode("utf-8", "ignore") directory = directory.rstrip('\n') self.fm.execute_console("cd " + directory)